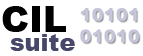
Field Stat
// First Pass
Visit scan = new Visit();
scan.Collectors.Register("App", new AppCollector());
scan.Collectors.Register("CallGraph", new CallGraphCollector());
scan.DoScan (m_Files, htAppBin, filters);
AppCollector appresult = (AppCollector)scan.Collectors["App"];
CallGraphCollector callgraph = (CallGraphCollector)scan.Collectors["CallGraph"];
...
public class AppCollector : AbstractCollector
{
...
public override void OnApplication(string application)
{
m_appResult = new AppResult(application);
m_AppDataList.Add(m_appResult);
}
public override void OnSystemMethodCall(MethodDefinition callingContext, MethodReference methodCall,
string callTypeName, string callMethodName)
{
IncrementByOne(m_appResult.SystemCallsHashtable, callMethodName);
}
}
CIL Diff
Diff API
Service | Description |
---|---|
GetMergedDifference | Get a new assembly with both the new and old version of code. This is useful for being able to test differences in versions and maintaining a persisted version. |
GetChangedCodeElements | Get just the types, methods, and properties that have changed between the two versions. |
GetChangeSummary | Get a text summary of the changes. |
GetChangePairs | Advanced. Get the relationships that have changed in a method body. For example, a different parameter to a method call, or a new method call is introduced to the body, or an assignment to a property is removed. |
Merge Properties for inspection.
MethodDefinition
Section | Type | Diff |
---|---|---|
Signature | New, Deleted | |
Body | New, Deleted, Modified | "Instruction:+1;-3;*4,5" |
ReturnType | Same, Different | |
Attributes | Same, Different | "Abstract; Assem; Compilercontrolled; FamANDAssem; Family; FamORAssem; Final; HasSecurity; HideBySig; MemberAccessMask; NewSlot; PInvokeImpl; Private; Public; RequireSecObject; ReuseSlot; RTSpecialName; SpecialName; Static; UnmanagedExport; Virtual; VtableLayoutMask" |
Properities | Same, Different | "CallingConvention; ExplicitThis; HasThis; ImplAttributes; IsVirtual; SecurityDeclarations; SemanticsAttributes; NoInlining" |
Overrides | Same, Different | "MethodReference:0,0;" |
Parameters/GenericParameters | Same, Different | "Name;in" |
CustomAttributes | Same, Different | "Return:+3;MethodDef:*4,4;Parameters:1:-2;GenParameters:0:+1" |
FieldDefinition
Section | Type | Diff |
---|---|---|
Signature | New, Deleted | |
Attributes | Same, Different | "Assembly; Compilercontrolled; FamANDAssem; Family; FamORAssem; FieldAccessMask; HasDefault; HasFieldMarshal; HasFieldRVA; InitOnly; Literal; NotSerialized; PInvokeImpl; Private; Public; RTSpecialName; SpecialName; Static" |
Properities | Same, Different | "FieldType;Constant;InitialValue" |
CustomAttributes | Same, Different | "Return:+3;MethodDef:*4,4;Parameters:1:-2;GenParameters:0:+1" |
PropertyDefinition
Section | Type | Diff |
---|---|---|
Signature | New, Deleted | |
Get/Set Method | (Handled in Method Definition) | |
Attributes | Same, Different | "HasDefault;RTSpecialName;SpecialName" |
Properities | Same, Different | "PropertyType" |
CustomAttributes | Same, Different | "Return:+3;MethodDef:*4,4;Parameters:1:-2;GenParameters:0:+1" |
TypeDefinition
Section | Type | Diff |
---|---|---|
Signature | New, Deleted | (should include Generic Parameters) |
Children | (in Fields,Properties,Events,Methods,Constructors,InnerTypes) | |
Attributes | Same, Different | "Abstract; AnsiClass; AutoClass; AutoLayout; BeforeFieldInit; Class; ClassSemanticMask; ExplicitLayout; HasSecurity; Import; Interface; LayoutMask; NestedAssembly; NestedFamANDAssem; NestedFamily; NestedFamORAssem; NestedPrivate; NestedPublic; NotPublic; Public; RTSpecialName; Sealed; SequentialLayout; Serializable; SpecialName; StringFormatMask, UnicodeClass; VisibilityMask" |
Properties | Same, Different | "BaseType; ClassSize; HasLayoutInfo; HasSecurity; IsClass; IsEnum; IsInterface; IsValueType; PackingSize; SecurityDeclarations" |
Interfaces | Same, Different | "TypeReference;TypeReference'" |